Generate A Dictionary With Keys With Empty Sets Python
- Python 3 Basic Tutorial
- Generate A Dictionary With Keys With Empty Sets Python Free
- Python Dictionary Keys Method
- Generate A Dictionary With Keys With Empty Sets Python Code
- Python Dictionary Key To List
- Dictionary With Keys Python
- Python 3 Advanced Tutorial
How to Create an Empty Set in Python. In this article, we show how to create an empty set in Python. First of all, what is a set in Python? A set is a collection of unique items. So, in a set, no items can be repeated. So if you want to create a list of items in Python, in which each item is unique, then you would create a set. Python Set Operations. Sets can be used to carry out mathematical set operations like union, intersection, difference and symmetric difference. We can do this with operators or methods. Mar 30, 2012 Dictionaries are the fundamental data structure in Python, and a key tool in any Python programmer’s arsenal. They allow O(1) lookup speed, and have been heavily optimized for memory overhead and lookup speed efficiency. Today I”m going to show you three ways of constructing a Python dictionary, as well as some additional tips and tricks. Jan 06, 2020 Welcome In this article, you will learn the fundamentals of Sets in Python. This is a very powerful built-in data type that you can use in your Python projects. We will explore:. What sets are and why they are relevant for your projects. How to create a set. How to check if an element is in a set. The difference between sets and frozensets.
Python Dictionary keys The keys method returns a view object that displays a list of all the keys in the dictionary.
- Python 3 Useful Resources
- Selected Reading
Each key is separated from its value by a colon (:), the items are separated by commas, and the whole thing is enclosed in curly braces. An empty dictionary without any items is written with just two curly braces, like this: {}.
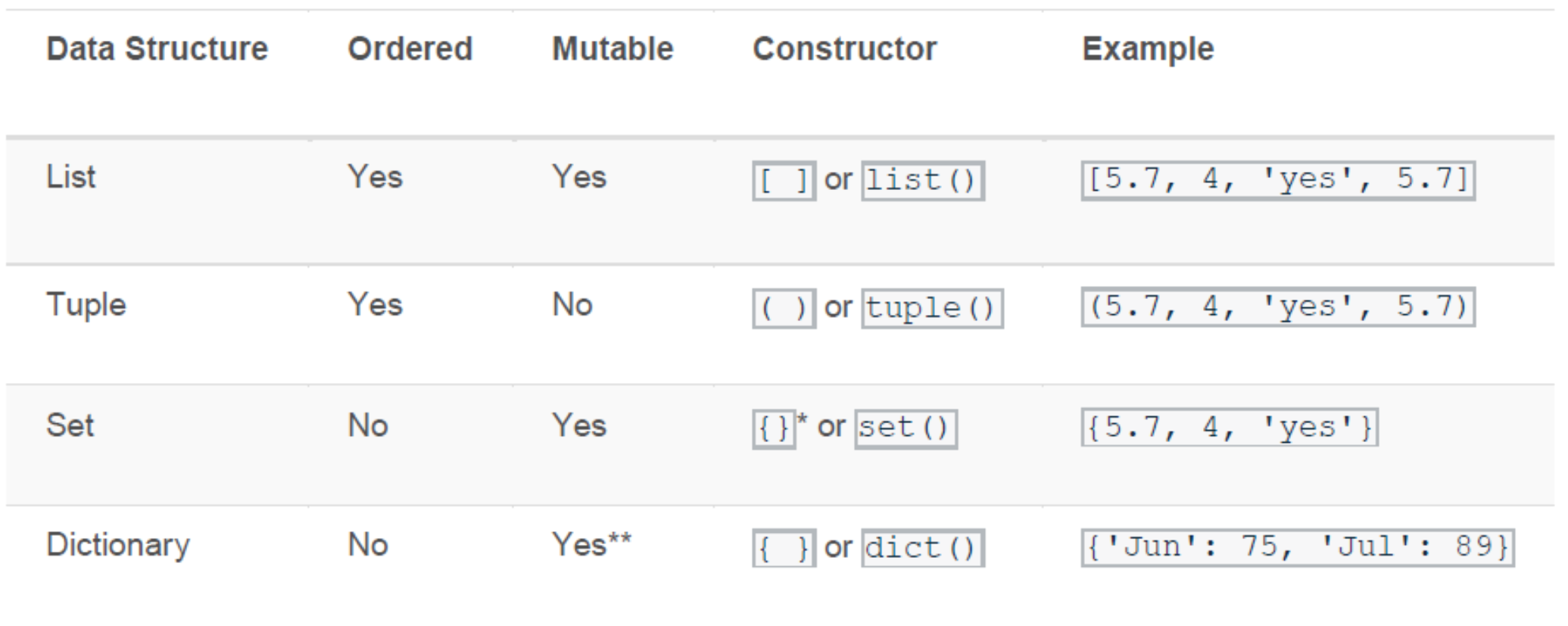
Keys are unique within a dictionary while values may not be. The values of a dictionary can be of any type, but the keys must be of an immutable data type such as strings, numbers, or tuples.
Accessing Values in Dictionary
To access dictionary elements, you can use the familiar square brackets along with the key to obtain its value. Following is a simple example −
When the above code is executed, it produces the following result −
If we attempt to access a data item with a key, which is not a part of the dictionary, we get an error as follows −
When the above code is executed, it produces the following result −
Updating Dictionary
You can update a dictionary by adding a new entry or a key-value pair, modifying an existing entry, or deleting an existing entry as shown in a simple example given below.
When the above code is executed, it produces the following result −
Generate A Dictionary With Keys With Empty Sets Python Free
Delete Dictionary Elements
You can either remove individual dictionary elements or clear the entire contents of a dictionary. You can also delete entire dictionary in a single operation.
To explicitly remove an entire dictionary, just use the del statement. Following is a simple example −
This produces the following result.
An exception is raised because after del dict, the dictionary does not exist anymore.
Note − The del() method is discussed in subsequent section.
Properties of Dictionary Keys
Dictionary values have no restrictions. They can be any arbitrary Python object, either standard objects or user-defined objects. However, same is not true for the keys.
There are two important points to remember about dictionary keys −
(a) More than one entry per key is not allowed. This means no duplicate key is allowed. When duplicate keys are encountered during assignment, the last assignment wins. For example −
When the above code is executed, it produces the following result −
(b) Keys must be immutable. This means you can use strings, numbers or tuples as dictionary keys but something like ['key'] is not allowed. Following is a simple example −
When the above code is executed, it produces the following result −
Built-in Dictionary Functions and Methods
Python includes the following dictionary functions −
Sr.No. | Function & Description |
---|---|
1 | cmp(dict1, dict2) No longer available in Python 3. |
2 | len(dict) Gives the total length of the dictionary. This would be equal to the number of items in the dictionary. |
3 | str(dict) Produces a printable string representation of a dictionary |
4 | type(variable) Returns the type of the passed variable. If passed variable is dictionary, then it would return a dictionary type. |
Python includes the following dictionary methods −
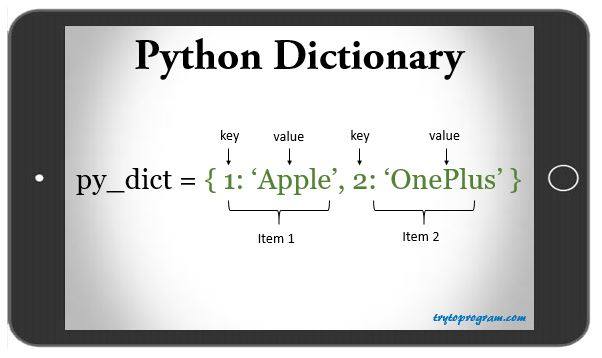
Sr.No. | Method & Description |
---|---|
1 | dict.clear() Online key generator for corel draw x7. Removes all elements of dictionary dict |
2 | dict.copy() Returns a shallow copy of dictionary dict |
3 | dict.fromkeys() Create a new dictionary with keys from seq and values set to value. |
4 | dict.get(key, default=None) For key key, returns value or default if key not in dictionary |
5 | dict.has_key(key) Removed, use the in operation instead. |
6 | dict.items() Returns a list of dict's (key, value) tuple pairs |
7 | dict.keys() Returns list of dictionary dict's keys |
8 | dict.setdefault(key, default = None) Similar to get(), but will set dict[key] = default if key is not already in dict |
9 | dict.update(dict2) Adds dictionary dict2's key-values pairs to dict |
10 | dict.values() Returns list of dictionary dict's values |
With Python, creating and using a dictionary is much like working with a list, except that you must now define a key and value pair. Here are the special rules for creating a key:
Python Dictionary Keys Method
The key must be unique. When you enter a duplicate key, the information found in the second entry wins — the first entry is simply replaced with the second.
The key must be immutable. This rule means that you can use strings, numbers, or tuples for the key. You can’t, however, use a list for a key.
You have no restrictions on the values you provide. A value can be any Python object, so you can use a dictionary to access an employee record or other complex data. The following steps help you understand how to use dictionaries better.
1Open a Python Shell window.
You see the familiar Python prompt.
2Type Colors = {“Sam”: “Blue”, “Amy”: “Red”, “Sarah”: “Yellow”} and press Enter.
Python creates a dictionary containing three entries with people’s favorite colors. Notice how you create the key and value pair. The key comes first, followed by a colon and then the value. Each entry is separated by a comma.
3Type Colors and press Enter.
You see the key and value pairs. However, notice that the entries are sorted in key order. A dictionary automatically keeps the keys sorted to make access faster, which means that you get fast search times even when working with a large data set. The downside is that creating the dictionary takes longer than using something like a list because the computer is busy sorting the entries.
4Type Colors[“Sarah”] and press Enter.
You see the color associated with Sarah, Yellow. Using a string as a key, rather than using a numeric index, makes the code easier to read and makes it self-documenting to an extent.
By making your code more readable, dictionaries save you considerable time in the long run (which is why they’re so popular). However, the convenience of a dictionary comes at the cost of additional creation time and a higher use of resources, so you have trade-offs to consider.
5Type Colors.keys( ) and press Enter.
The dictionary presents a list of the keys it contains. You can use these keys to automate access to the dictionary.
6Type the following code (pressing Enter after each line and pressing Enter twice after the last line):
The example code outputs a listing of each of the user names and the user’s favorite color. Using dictionaries can make creating useful output a lot easier. The use of a meaningful key means that the key can easily be part of the output.
7Type Colors[“Sarah”] = “Purple” and press Enter.
The dictionary content is updated so that Sarah now likes Purple instead of Yellow.
Generate A Dictionary With Keys With Empty Sets Python Code
8Type Colors.update({“Harry”: “Orange”}) and press Enter.
Windows 10 product key torrents. A new entry is added to the dictionary.
9Place your cursor at the end of the third line of the code you typed in Step 6 and press Enter.
The editor creates a copy of the code for you. This is a time-saving technique that you can use in the Python Shell when you experiment while using code that takes a while to type. Even though you have to type it the first time, you have no good reason to type it the second time.
Python Dictionary Key To List
10Press Enter twice.
Notice that Harry is added in sorted order. In addition, Sarah’s entry is changed to the color Purple.
11Type del Colors[“Sam”] and press Enter.
Python removes Sam’s entry from the dictionary.
12Repeat Steps 9 and 10.
You verify that Sam’s entry is actually gone.
13Type len(Colors) and press Enter.
Dictionary With Keys Python
The output value of 3 verifies that the dictionary contains only three entries now, rather than 4.
14Type Colors.clear( ) and press Enter. Then, Type len(Colors) and press Enter.
Python reports that Colors has 0 entries, so the dictionary is now empty.