Python Generate Random Aes Key
I'm trying to build two functions using PyCrypto that accept two parameters: the message and the key, and then encrypt/decrypt the message. I found several links on the web to help me out, but each one of them has flaws. This only works because the 'mysecretpassword' is 16 bytes. If it were a different (not dividable by 16) amount of bytes you'd get 'ValueError: AES key must be either 16, 24, or 32 bytes long'.
Encrypt & Decrypt using PyCrypto AES 256 (6)
I spent a little bit of time last night and this morning trying to find some examples for AES encryption using Python and PyCrypto.To my surprise, I had quite a difficult time finding an example of how to do it!
Another take on this (heavily derived from solutions above) but
Sep 26, 2019 an example of symmetric encryption in python using a single known secret key - utilizes AES from PyCrypto library - AESexample.py. # generate a random secret key with the decided key length. # use the decoded secret key to create a AES cipher: cipher = AES. New (secretkey). How can I securely convert a “string” password to a key used in AES? Ask Question Asked 6. Generate a random 128-bit key (k1), a random 128-bit IV, and a random. But maybe you don't), then you could skip the steps involving k1, and use k2 as your AES key and k3 to verify it. You may also find it useful to generate another key for. # It should typically be random data, or bytes that resemble random data such # as the hash of a password. # The number of bytes in the secret key defines the bit-strength of an encryption # algorithm. For example, AES with a 32-byte key is 256-bit AES. Most algorithms # define restrictions on key sizes.
- uses null for padding
- does not use lambda (never been a fan)
tested with python 2.7 and 3.6.5
I'm trying to build two functions using PyCrypto that accept two parameters: the message and the key, and then encrypt/decrypt the message.
Windows 7 professional key code generator reviews. I found several links on the web to help me out, but each one of them has flaws:
This one at codekoala uses os.urandom, which is discouraged by PyCrypto.
Moreover, the key I give to the function is not guaranteed to have the exact length expected. What can I do to make that happen ?
Also, there are several modes, which one is recommended? I don't know what to use :/
Finally, what exactly is the IV? Can I provide a different IV for encrypting and decrypting, or will this return in a different result?
Here's what I've done so far:
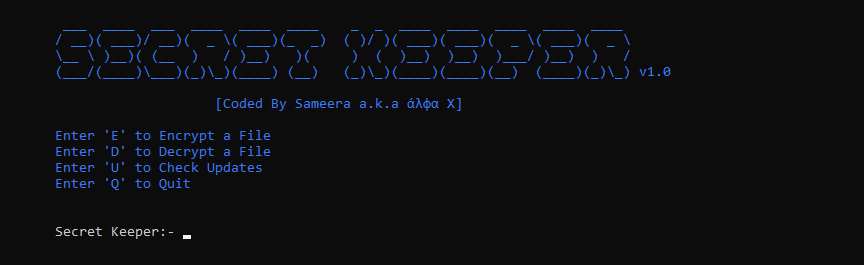
For someone who would like to use urlsafe_b64encode and urlsafe_b64decode, here are the version that're working for me (after spending some time with the unicode issue)
# Inspired from https://pythonprogramming.net/encryption-and-decryption-in-python-code-example-with-explanation/ |
# PyCrypto docs available at https://www.dlitz.net/software/pycrypto/api/2.6/ |
fromCrypto.CipherimportAES |
importbase64, os |
defgenerate_secret_key_for_AES_cipher(): |
# AES key length must be either 16, 24, or 32 bytes long |
AES_key_length=16# use larger value in production |
# generate a random secret key with the decided key length |
# this secret key will be used to create AES cipher for encryption/decryption |
secret_key=os.urandom(AES_key_length) |
# encode this secret key for storing safely in database |
encoded_secret_key=base64.b64encode(secret_key) |
returnencoded_secret_key |
defencrypt_message(private_msg, encoded_secret_key, padding_character): |
# decode the encoded secret key |
secret_key=base64.b64decode(encoded_secret_key) |
# use the decoded secret key to create a AES cipher |
cipher=AES.new(secret_key) |
# pad the private_msg |
# because AES encryption requires the length of the msg to be a multiple of 16 |
padded_private_msg=private_msg+ (padding_character* ((16-len(private_msg)) %16)) |
# use the cipher to encrypt the padded message |
encrypted_msg=cipher.encrypt(padded_private_msg) |
# encode the encrypted msg for storing safely in the database |
encoded_encrypted_msg=base64.b64encode(encrypted_msg) |
# return encoded encrypted message |
returnencoded_encrypted_msg |
defdecrypt_message(encoded_encrypted_msg, encoded_secret_key, padding_character): |
# decode the encoded encrypted message and encoded secret key |
secret_key=base64.b64decode(encoded_secret_key) |
encrypted_msg=base64.b64decode(encoded_encrypted_msg) |
# use the decoded secret key to create a AES cipher |
cipher=AES.new(secret_key) |
# use the cipher to decrypt the encrypted message |
decrypted_msg=cipher.decrypt(encrypted_msg) |
# unpad the encrypted message |
unpadded_private_msg=decrypted_msg.rstrip(padding_character) |
# return a decrypted original private message |
returnunpadded_private_msg |
####### BEGIN HERE ####### |
private_msg='' |
Lorem ipsum dolor sit amet, malis recteque posidonium ea sit, te vis meliore verterem. Duis movet comprehensam eam ex, te mea possim luptatum gloriatur. Modus summo epicuri eu nec. Ex placerat complectitur eos. |
'' |
padding_character='{' |
secret_key=generate_secret_key_for_AES_cipher() |
encrypted_msg=encrypt_message(private_msg, secret_key, padding_character) |
decrypted_msg=decrypt_message(encrypted_msg, secret_key, padding_character) |
print' Secret Key: %s - (%d)'% (secret_key, len(secret_key)) |
print'Encrypted Msg: %s - (%d)'% (encrypted_msg, len(encrypted_msg)) |
print'Decrypted Msg: %s - (%d)'% (decrypted_msg, len(decrypted_msg)) |
commented Jan 18, 2019 • edited
edited
Random Numbers Python
See also https://gist.github.com/btoueg/f71b62f456550da42ea9f4a4bd907d21 for an example using cryptography |